In the previous post, we explained how we came to implement the new 2-dimensional grid system. This was needed for our plans to make the levels of Highrisers procedurally generated.
So, now we had a nice and fancy grid, and we knew what area was covered by each specific item; we called that the slot size, measured in x and z direction:
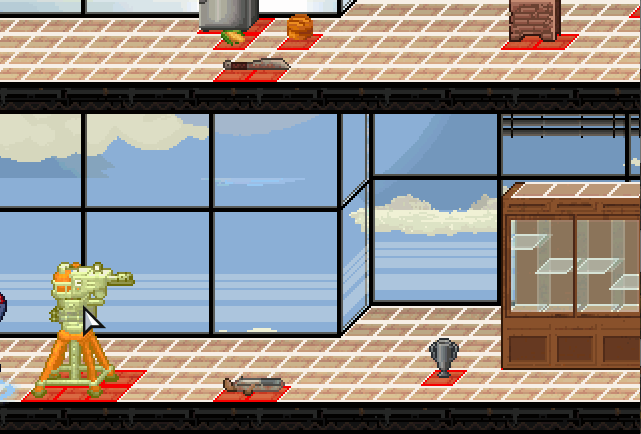
So, in this pic the trophy has a slotsize of (1, 1), the crossbow needs (2, 1) and the turret requires an area of (3, 2).
What next? In procedural generation, every time a player enters a new level, you want him to feel as if he has never seen this arrangement before. This is reached through high levels of randomization – the more randomness and chaos you have, the lower the risk of a player saying: “Wait a second… I’ve seen that before.”
On the other hand, every environment, and especially a man-made environment like a building, there is a certain order that a player would expect. Furniture is placed and arranged in a certain way that feels proper – nobody places his fridge in front of a window; chairs are normally placed facing towards a table; books are placed in shelves, and so on.
When aiming for minimum risk of rediscovering the same set of objects, we could let a random generator run over the available objects, let it place them wherever there was space, and then see what we get. It would be a happy mess.
In order to get a certain grade of order, we thought of tagging certain objects to be belonging to a certain type (like “tools” or “kitchen stuff” and the like); and we could also define certain rules like “put furniture close to the walls”; but we still wouldn’t end up with what we wanted.
For a believable building, we needed more order, so we decided to hand-craft certain small groups of objects and furniture that were arranged in a way that makes sense and are visually pleasing, and then let those be placed in the levels in smart ways.
For that, we needed an tool to assemble and arrange those groups. We came to call it the Furniture Group Editor:
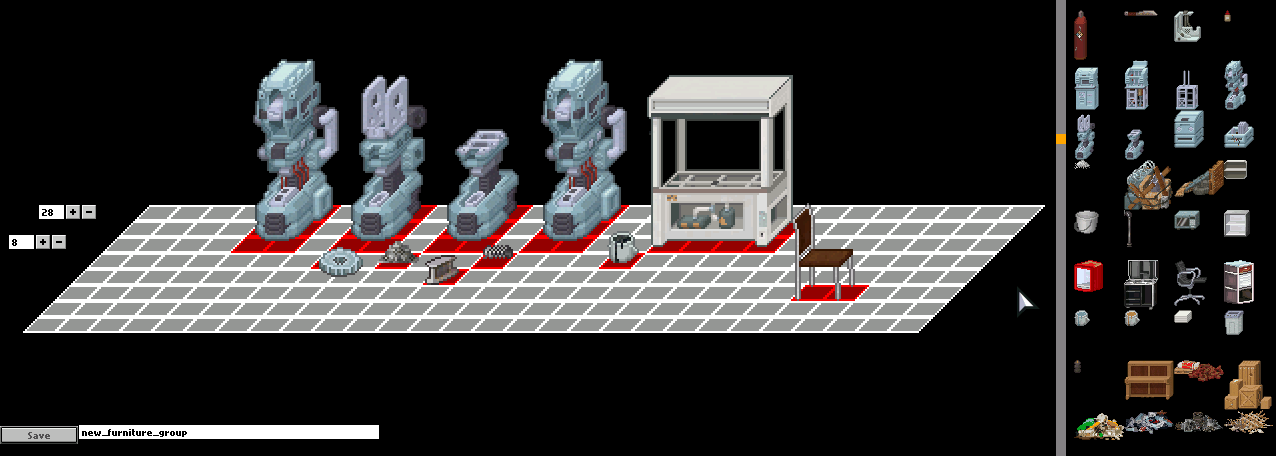
As you can see, it enabled us to position objects, to cycle through object variants and to adjust parameters like directional facing. Progress in object modularization also made it possible to create a vast amount of furniture looks with a minimum effort:
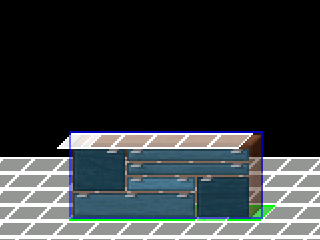
So we’ve created a selection of groups, we’ve tagged them so that a
floor would have a consistent theme, and we defined certain rules of how
to find positions for placement.
These are some of our current results:
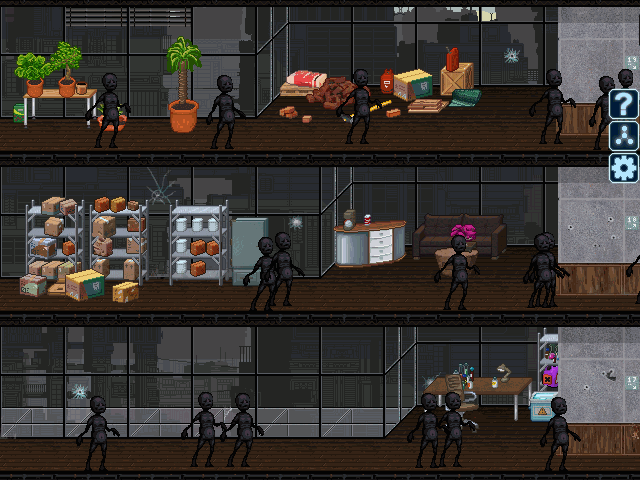
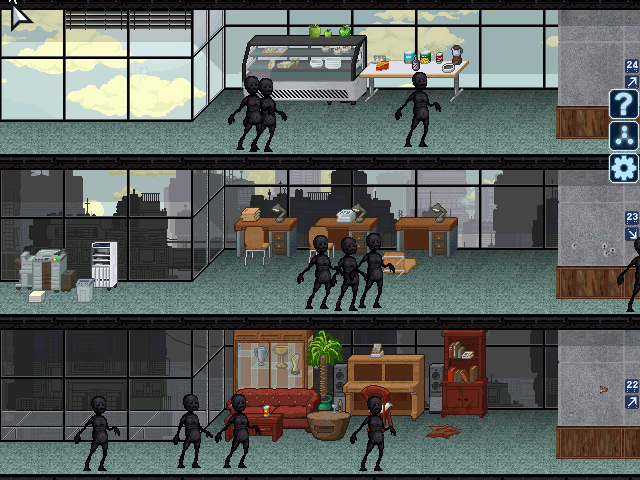
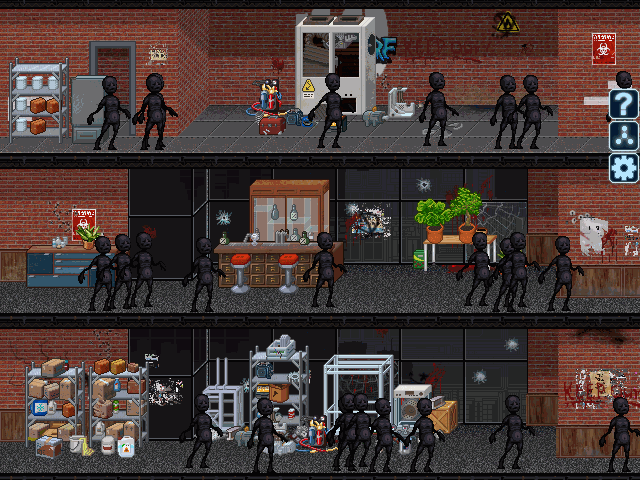
So, what’s next? If you look closely, you can still see the imperfections of the current approach.
There are groups that are repeated often; the reason for that is that we only have a limited number of groups in our collection yet.
Then, there is the issue that groups are static, which makes it very obvious to recognize them again – if there is the exact same dresser with the exact same aloe plant on top, people will recognize it. But if next time the dresser is reused, there are books on them, or clothes, or maybe nothing – then it gets harder to see the pattern.
And finally, there is the question of positioning. Currently, the algorithm is still quite dumb; it identifies corners of rooms and then tries to place groups in those. Then, if there is still space in between, it tries to fill them.
Improvements here could create a more dynamic, more natural object distribution.
We’ll keep you updated with what we come up with!